KittEncode: Cute Cat-Themed Shellcode Encoder
KittEncode is a unique project that transforms shellcode into cat-themed words, making binary code more approachable and fun to understand.
Core Concepts
- Shellcode: A small piece of machine code that can be injected into a running program
- Nibble: Half a byte, or 4 bits - this is our basic unit of conversion
Implementation
words = ["floof", "purrito", "meow", "blepp", "boop", "zoomies", "mlem", "loaf",
"chonk", "nap", "catnip", "whisker", "paws", "tail", "mittens", "kitteh"]
def encode_shellcode(shellcode):
encoded = []
for byte in shellcode:
high_nibble = (byte >> 4) & 0x0F # Get first 4 bits
low_nibble = byte & 0x0F # Get last 4 bits
encoded.append(f"{words[high_nibble]}-{words[low_nibble]}")
return " ".join(encoded)
How the Encoding Works
- Defines 16 cat-themed words (one for each 4-bit value 0-15)
- Processes shellcode byte by byte
- Splits each byte into two nibbles using bitwise operations
- Uses nibbles as indices to select cat words
- Joins word pairs with hyphens
Example Usage
Original shellcode: \x90\x50\x90
Encoded output: loaf-meow zoomies-floof loaf-meow
Each byte is transformed into a pair of cat words, making the shellcode appear harmless and cute.
Decoding Process
def decode_shellcode(encoded):
word_to_nibble = {word: i for i, word in enumerate(words)}
decoded = bytearray()
for pair in encoded.split():
high, low = pair.split('-')
byte = (word_to_nibble[high] << 4) | word_to_nibble[low]
decoded.append(byte)
return bytes(decoded)
Decoding Steps
- Creates a reverse lookup dictionary for cat words
- Splits encoded string into word pairs
- Converts words back to nibble values
- Combines nibbles to reconstruct original bytes
- Returns the original shellcode ready for execution
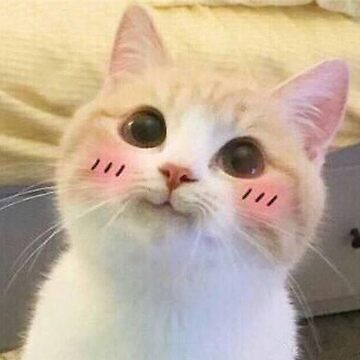